Getting your API keys
Learn how to obtain your API keys and understand the supported authentication strategies for securely accessing Hume APIs.
API keys
Each Hume account is provisioned with an API key and Secret key. These keys are accessible from the Hume Portal.
- Sign in: Visit the Hume Portal and log in, or create an account.
- View your API keys: Navigate to the API keys page to view your keys.
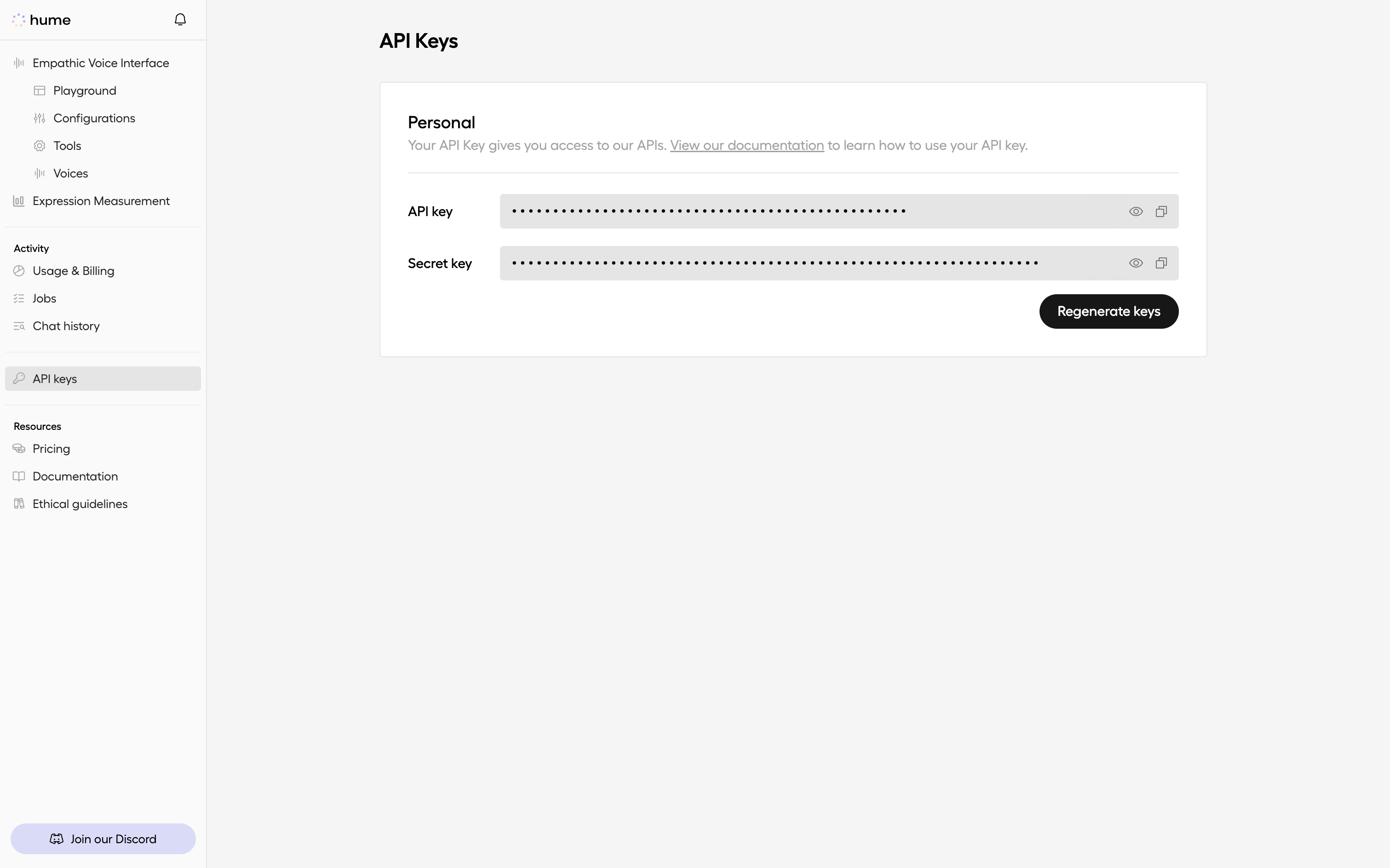
Authentication strategies
Hume APIs support two authentication strategies:
-
API key strategy: Use API key authentication for making server-side requests. API key authentication allows you to make authenticated requests by supplying a single secret using the
X-Hume-Api-Key
header. Do not expose your API key in client-side code. All Hume APIs support this authentication strategy. -
Token strategy: Use Token authentication for making client-side requests. With Token authentication you first obtain a temporary access token by making a server-side request first, and use the access token when making client-side requests. This allows you to avoid exposing the API key to the client. Access tokens expire after 30 minutes, and you must obtain a new one. Today, only our Empathic Voice Interface (EVI) supports this authentication strategy.
API key authentication
To use API key authentication on REST API endpoints, include the API key in the X-Hume-Api-Key
request header.
For WebSocket endpoints, include the API key as a query parameter in the URL.
Token authentication
To use Token authentication you must first obtain an Access Token from the POST /oauth2-cc/token
endpoint.
This is a unique endpoint that uses the “Basic” authentication scheme, with your API key as the username and the Secret key as the password. This means you must concatenate your API key and Secret key, separated by a colon (:
), base64 encode this value, and then put the result in the Authorization
header of the request, prefixed with Basic
.
You must also supply the grant_type=client_credentials
parameter in the request body.
On the client side, open an authenticated websocket by including the access token as a query parameter in the URL.
Or, make a REST request by including the access token in the Authorization
header.
The Hume Python and TypeScript SDKs will use the API key authentication strategy if you provide only the API key, but will use the Token authentication strategy if you provide both the API key and Secret key.
Regenerating API keys
API keys can be regenerated by clicking the Regenerate keys button on the API keys page. This permanently invalidates the current keys, requiring you to update any applications using them.
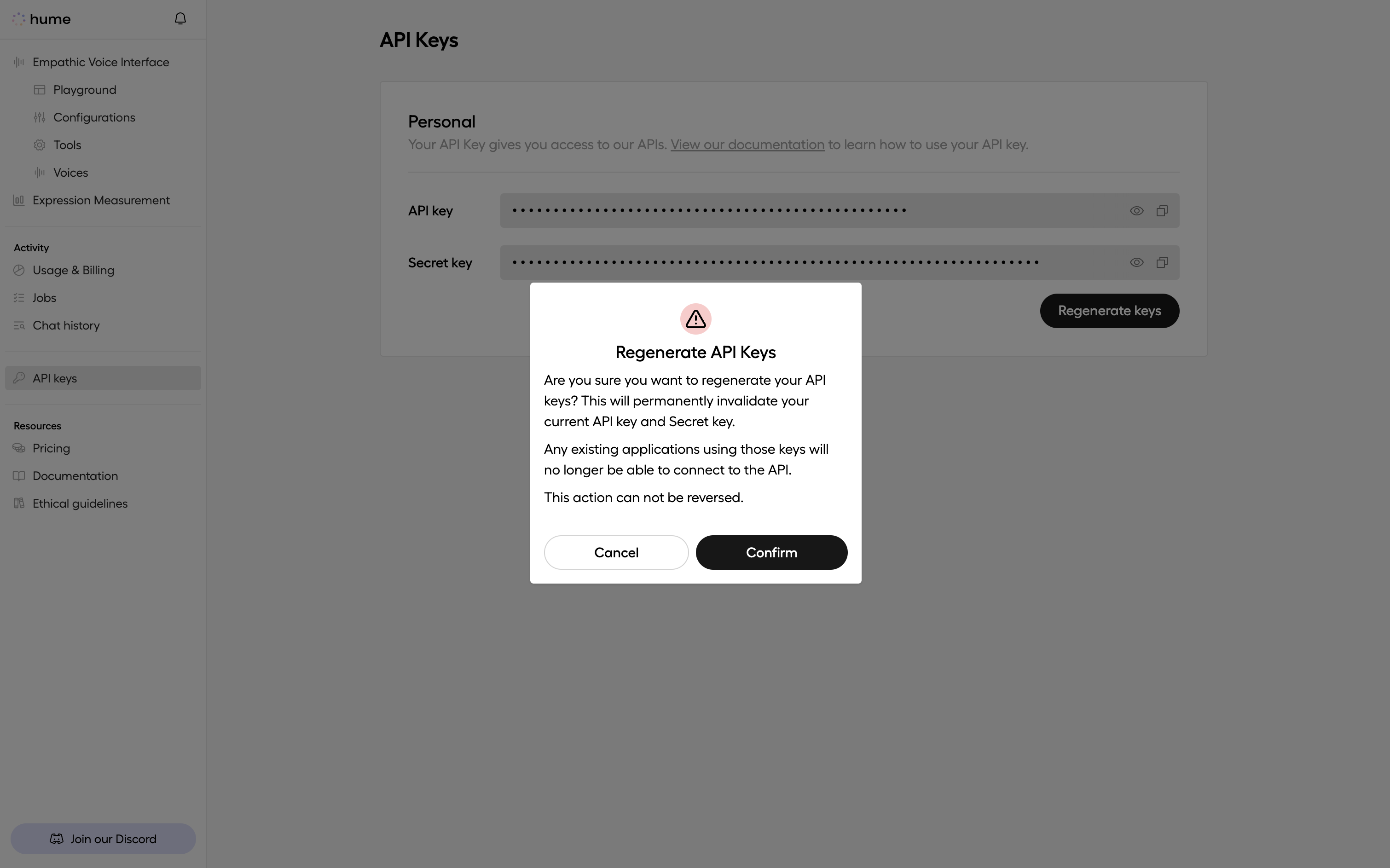