Tool Use
Guide to utilize function calling with EVI.
EVI simplifies the integration of external APIs through function calling. Developers can integrate custom functions that are invoked dynamically based on the user’s input, enabling more useful conversations. There are two key concepts for using function calling with EVI: Tools and Configurations (Configs):
-
Tools are resources that EVI uses to do things, like search the web or call external APIs. For example, tools can check the weather, update databases, schedule appointments, or take actions based on what occurs in the conversation. While the tools can be user-defined, Hume also offers natively implemented tools, like web search, which are labeled as “built-in” tools.
-
Configurations enable developers to customize an EVI’s behavior and incorporate these custom tools. Setting up an EVI configuration allows developers to seamlessly integrate their tools into the voice interface. A configuration includes prompts, user-defined tools, and other settings.
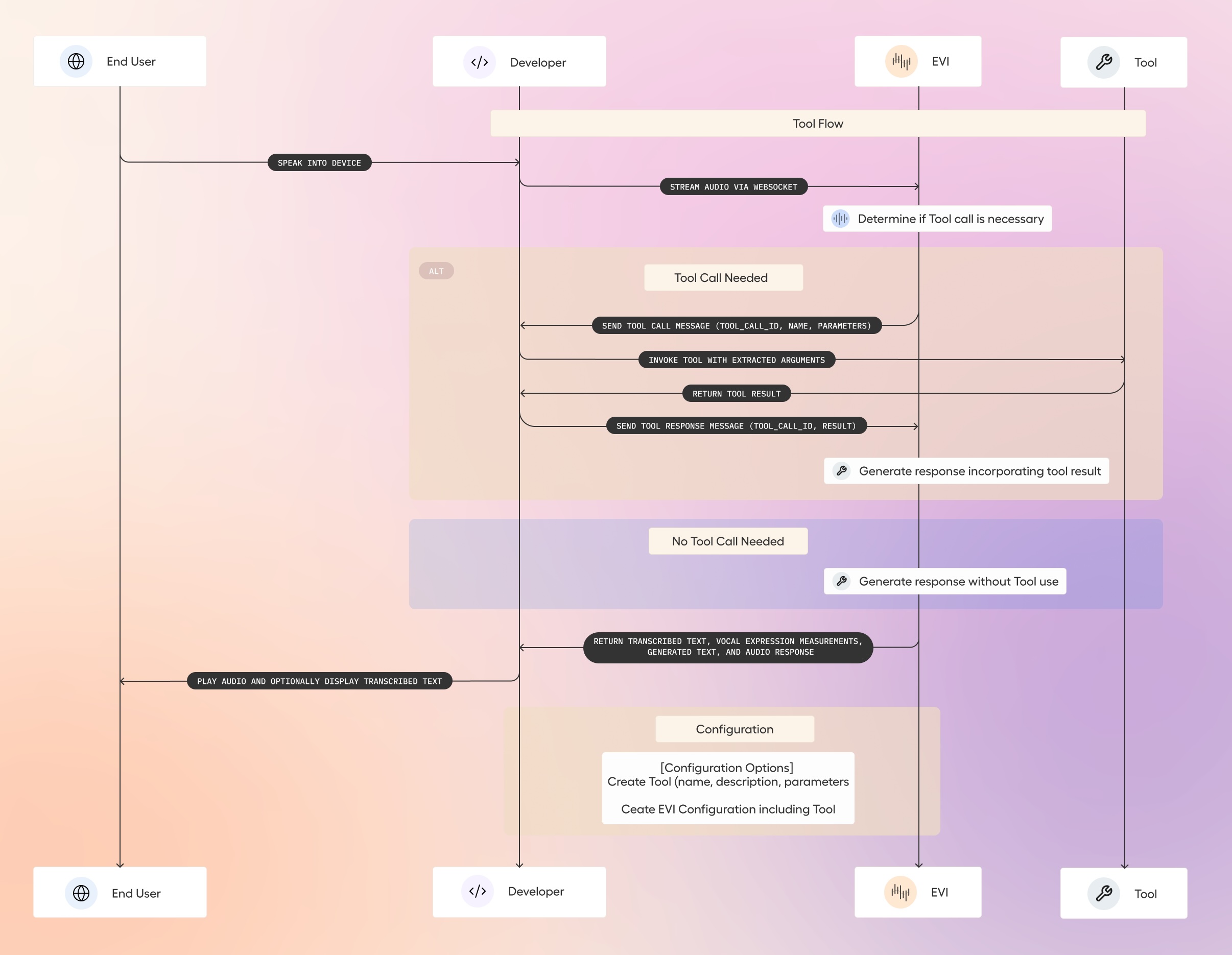
Currently, our function calling feature only supports OpenAI and Anthropic models. For the best results, we suggest choosing a fast and intelligent LLM that performs well on function calling benchmarks. On account of its speed and intelligence, we recommend Claude 3.5 Haiku as the supplemental LLM in your EVI configuration when using tools. Function calling is not available if you are using your own custom language model. We plan to support more function calling LLMs in the future.
The focus of this guide is on creating a Tool and a Configuration that allows EVI to use the Tool. Additionally, this guide details the message flow of function calls within a session, and outlines the expected responses when function calls fail. Refer to our Configuration Guide for detailed, step-by-step instructions on how to create and use an EVI Configuration.
Explore these sample projects to see how Tool use can be implemented in TypeScript, Next.js, and Python.
Setup
For EVI to leverage tools or call functions, a configuration must be created with the tool’s definition. Our step-by-step guide below walks you through creating a tool and adding it to a configuration, using either a no-code approach through our Portal or a full-code approach through our API.
No code
Full code
Create a Tool
We will first create a Tool with a specified function. In this example, we will create a tool for getting the weather. In the Portal, navigate to the EVI Tools page. Click the Create tool button to begin.
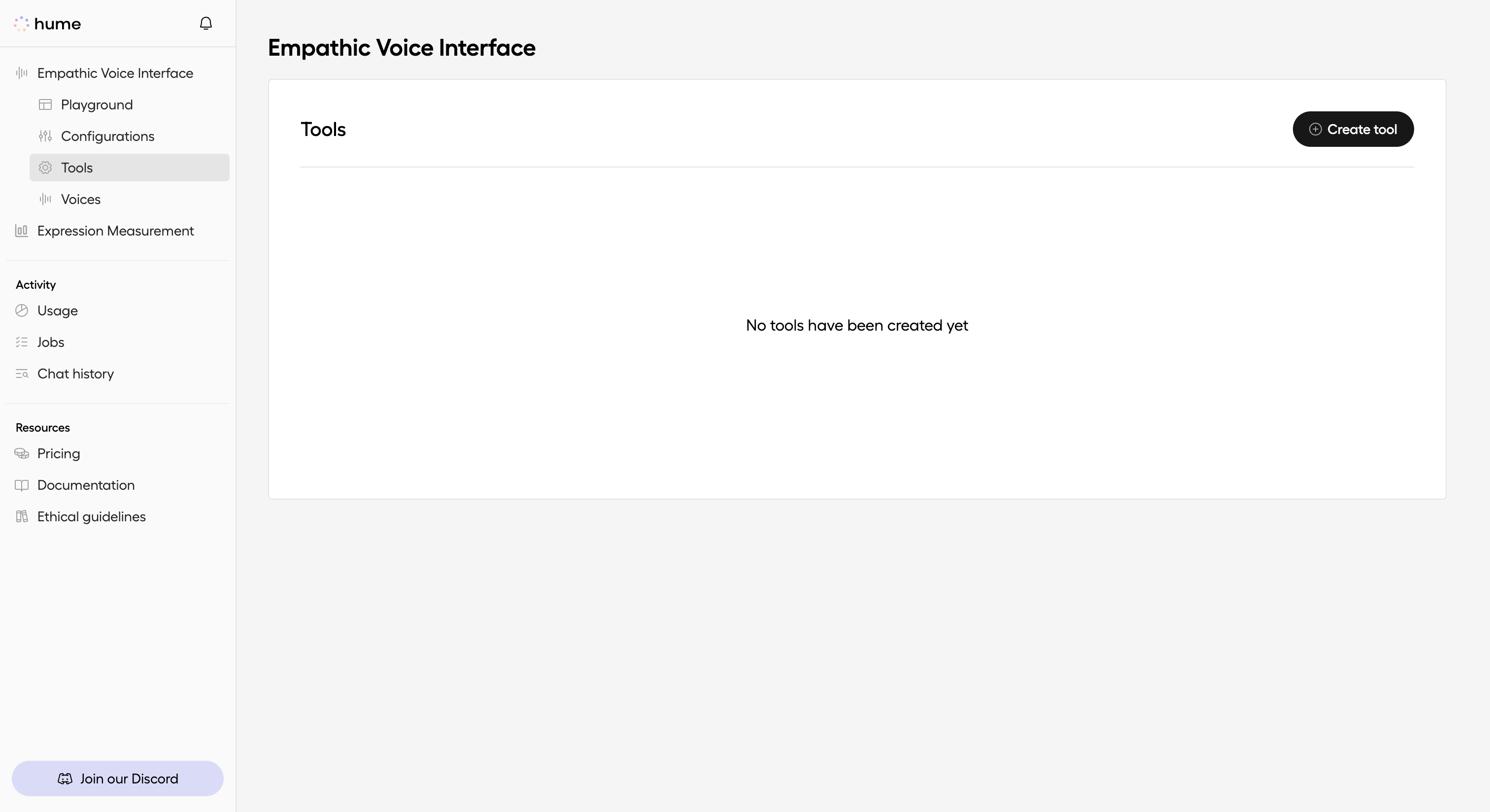
Fill in Tool details
Next, we will fill in the details for a weather tool named get_current_weather
. This tool fetches the current
weather conditions in a specified location and reports the temperature in either Celsius or Fahrenheit. We can
establish the tool’s behavior by completing the following fields:
- Name: Specify the name of the function that the language model will invoke. Ensure it begins with a lowercase letter and only contains letters, numbers, or underscores.
- Description: Provide a brief description of what the function does.
- Parameters: Define the function’s input parameters using a JSON schema.
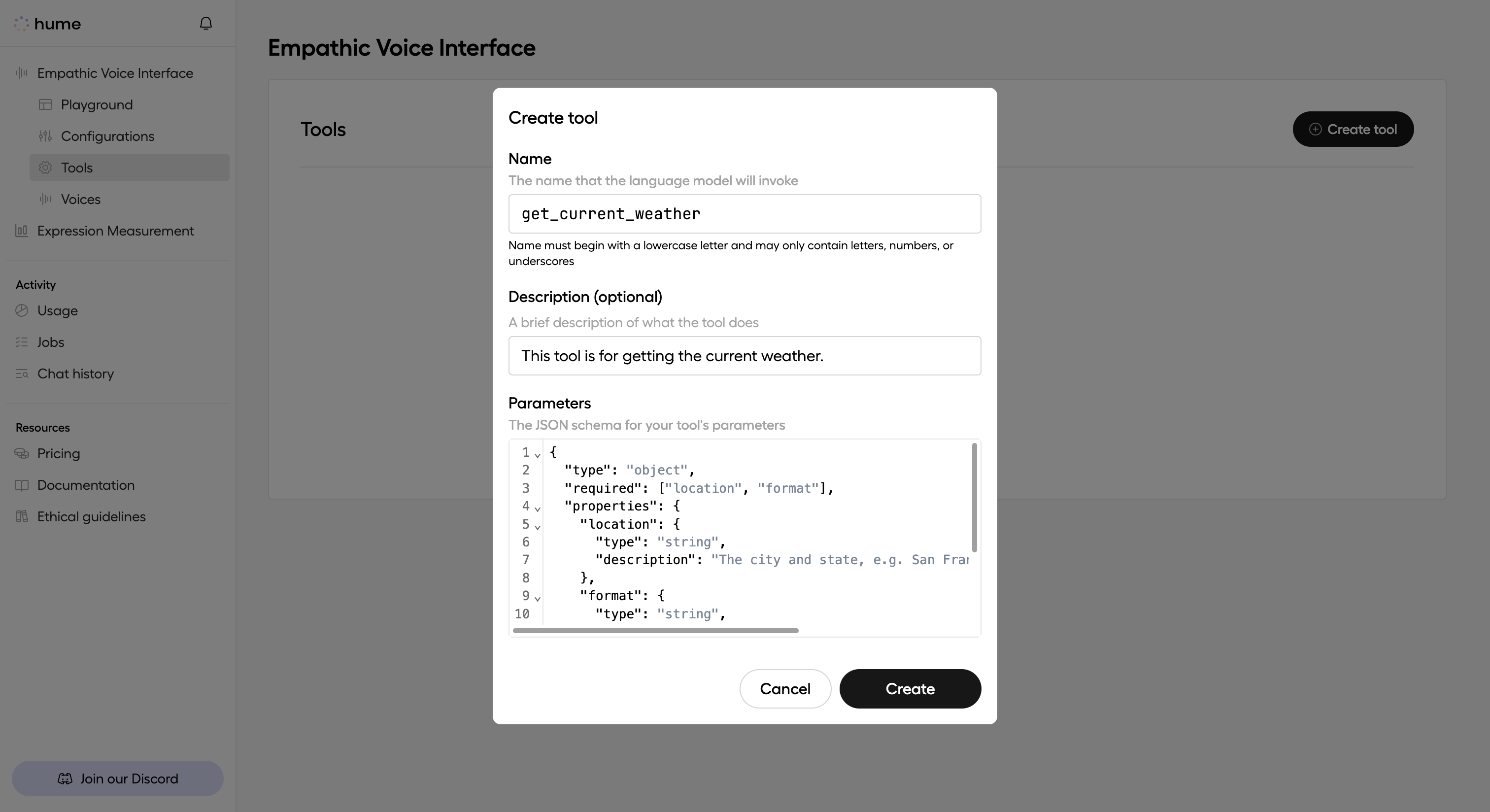
The JSON schema defines the expected structure of a function’s input parameters. Here’s an example JSON schema we can use for the parameters field of a weather function:
Create a Configuration
Next, we will create an EVI Configuration called Weather Assistant Config. This configuration will utilize the
get_current_weather
Tool created in the previous step. See our
Configuration guide for step-by-step
instructions on how to create a configuration. During the Set up LLM step, remember to select an Anthropic or
OpenAI model for tool use support.
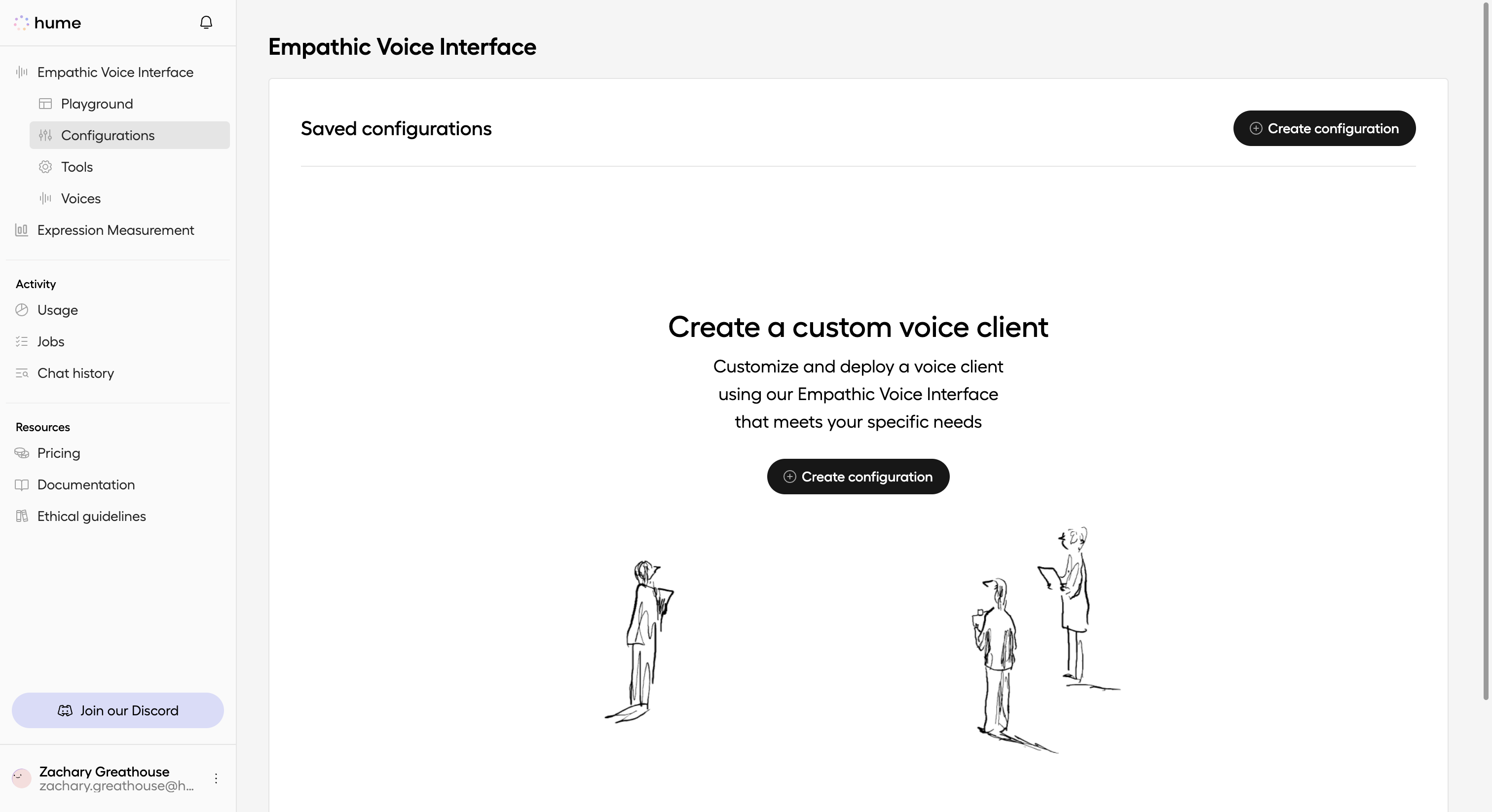
Add Tool to Configuration
Finally, we will specify the get_current_weather
Tool in the Weather Assistant Config. Navigate to the Tools
section of the EVI Config details page. Click the Add button to add a function to your configuration.
Since we have already created a get_current_weather
Tool in previous steps, we can simply select Add existing
tool… from the dropdown to specify it.
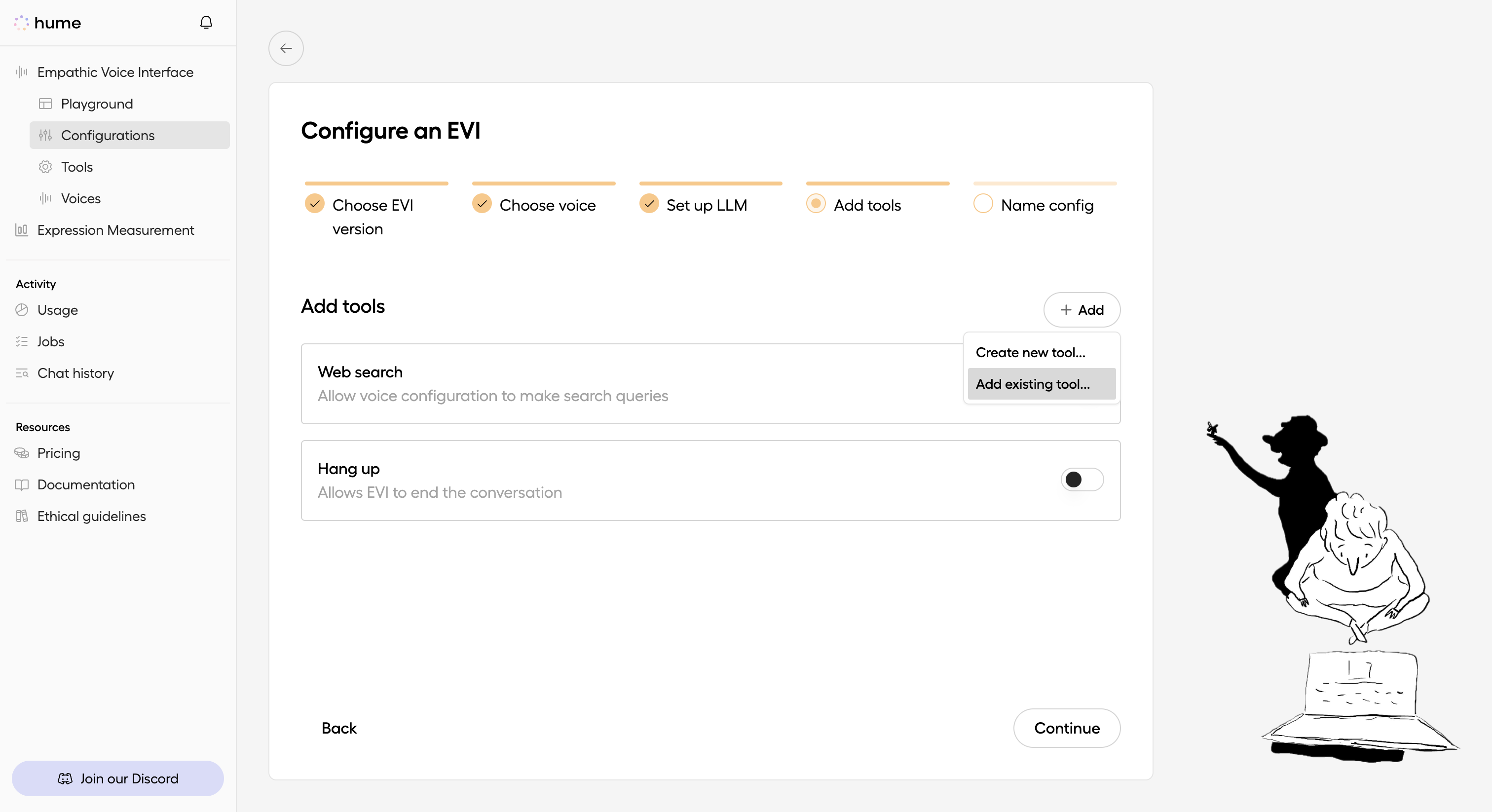
Select the tool to add get_current_weather
to your configuration, then complete the remaining steps to create the
configuration.
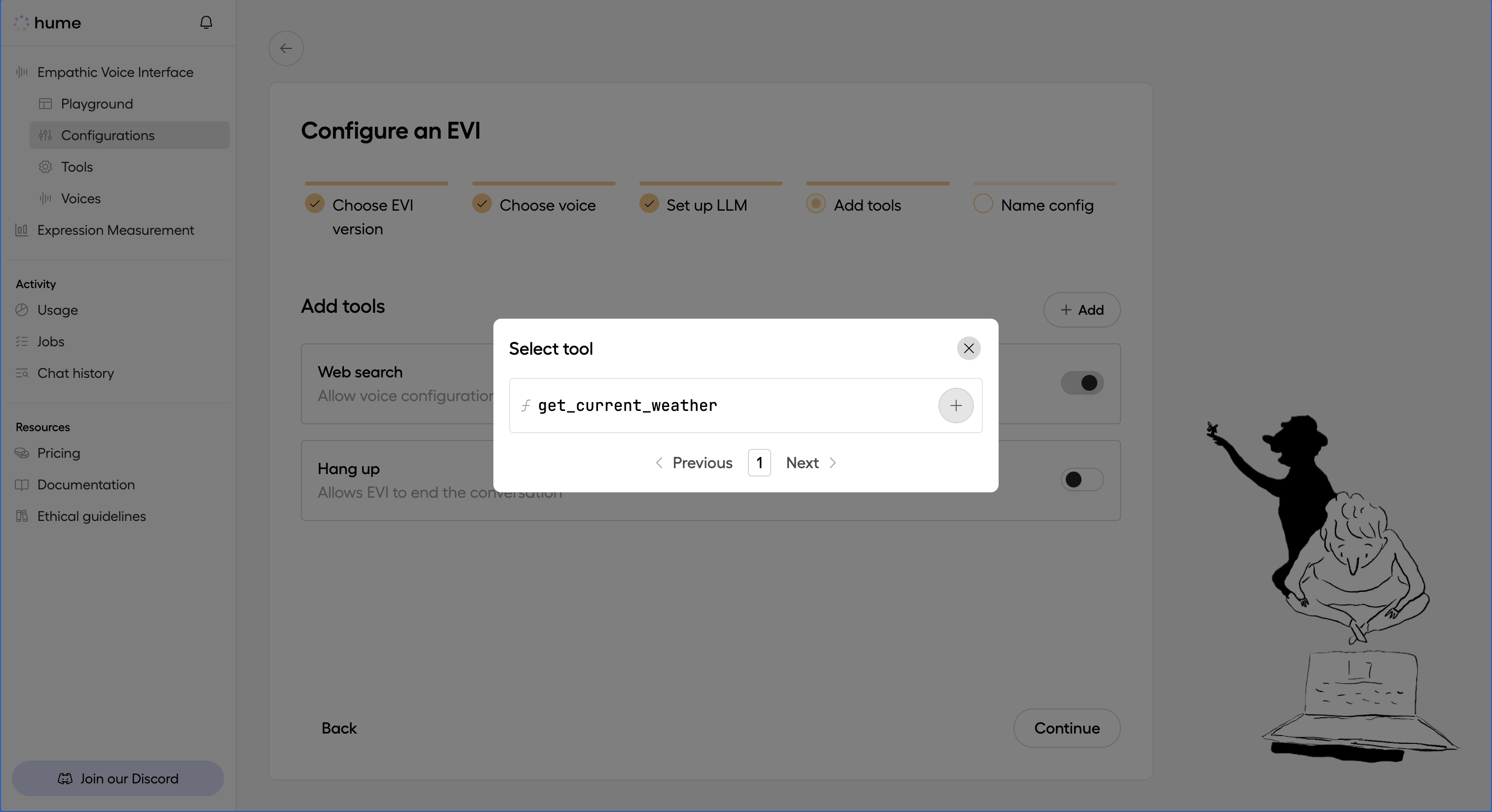
Function calling
In this section, we will go over the end-to-end flow of a function call within a chat session. This flow will be predicated on having specified the Weather Assistant Config when establishing a connection with EVI. See our Configuration Guide for details on how to apply your configuration when connecting.
Check out the TypeScript and Python example projects for complete implementations of the weather Tool you’ll build in this tutorial.
Define a function
We must first define a function for your Tool. This function will take the same parameters as those specified during your Tool’s creation.
For this tutorial, we will define a function that calls a weather API (e.g., the
Geocoding API) to retrieve the weather for a designated city in a specified format. This
weather function will accept location
and format
as its parameters.
See the code below for a sample implementation:
Instead of calling a weather API, you can hardcode a return value like 75F
as a means to quickly test for the sake
of this tutorial.
EVI signals function call
Once EVI is configured with your Tool, it will automatically infer when to signal a function call within a chat
session. With EVI configured to use the get_current_weather
Tool, we can now ask it: “what is the weather in New
York?”
Let’s try it out in the EVI Playground.
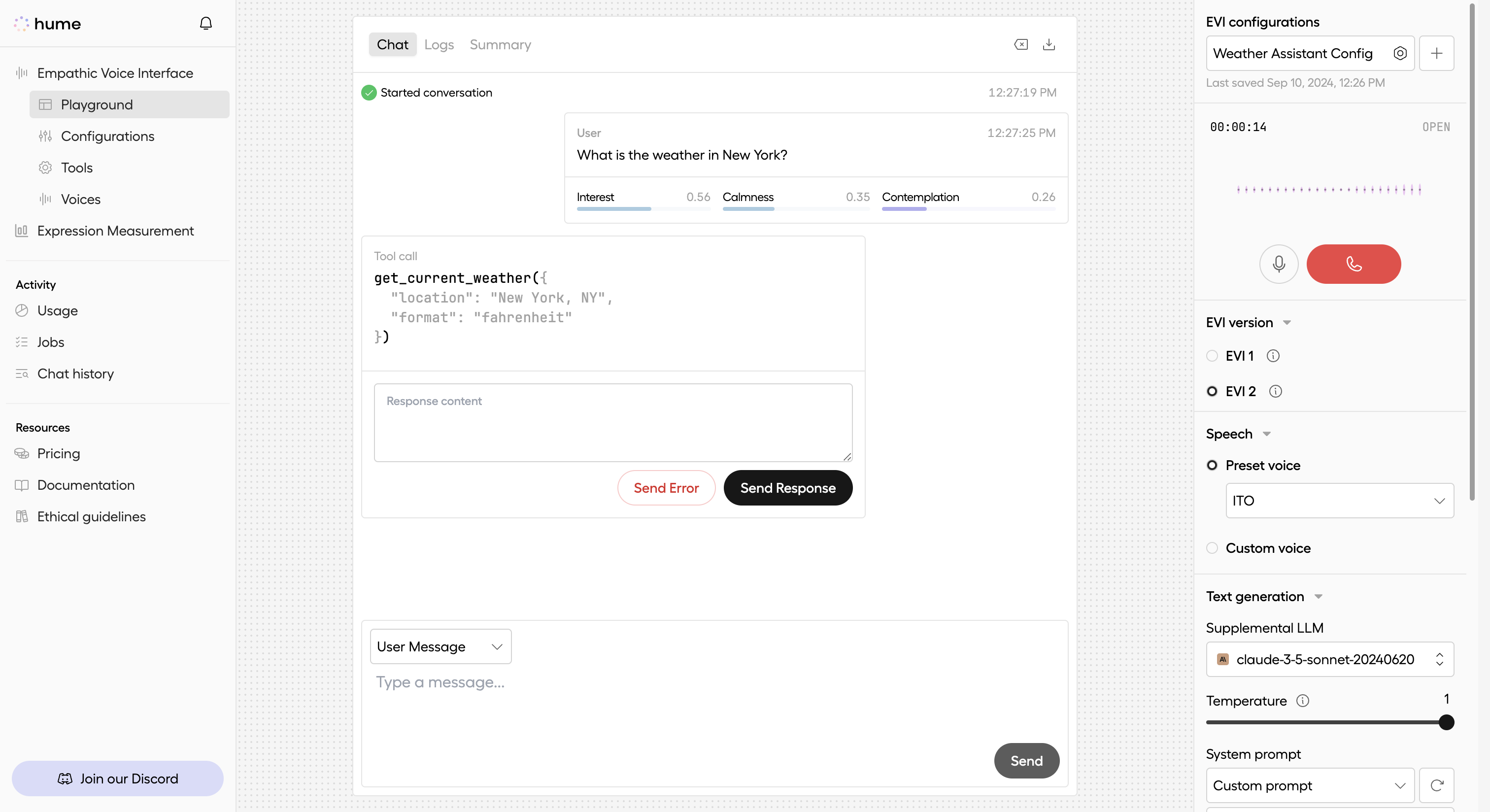
We can expect EVI to respond with a User Message and a Tool Call message:
Currently, EVI does not support parallel function calling. Only one function call can be processed at a time.
Extract arguments from Tool Call message
Upon receiving a Tool Call message from EVI, we will parse the parameters and extract the arguments.
The code below demonstrates how to extract the location
and format
arguments, which the user-defined fetch weather function is expecting, from a received Tool Call message.
Invoke function call
Next, we will pass the extracted arguments into the previously defined fetch weather function. We will capture the return value to send back to EVI:
Send function call result
Upon receiving the return value of your function, we will send a Tool Response message containing the result. The specified tool_call_id
must match the one received in
the Tool Call message from EVI:
Let’s try it in the EVI Playground. Enter the return value of your function in the input field below the Tool Call message, and click Send Response. In practice, you will use the actual return value from your function call. However, for demonstration purposes, we will assume a return value of “75F”.
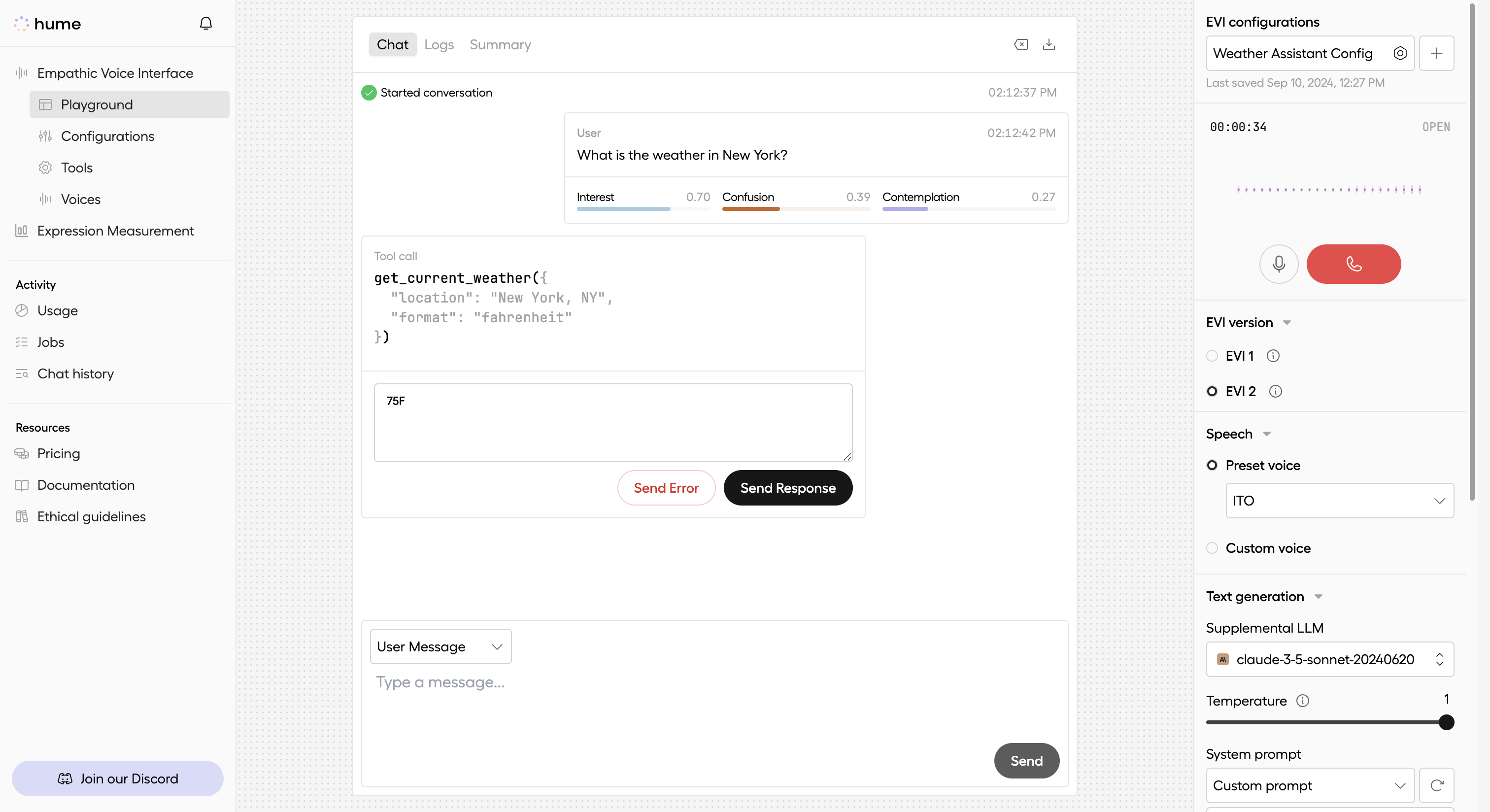
EVI responds
After the interface receives the Tool Response message, it will then send an Assistant Message containing the response generated from the reported result of the function call:
See how it works in the EVI Playground.
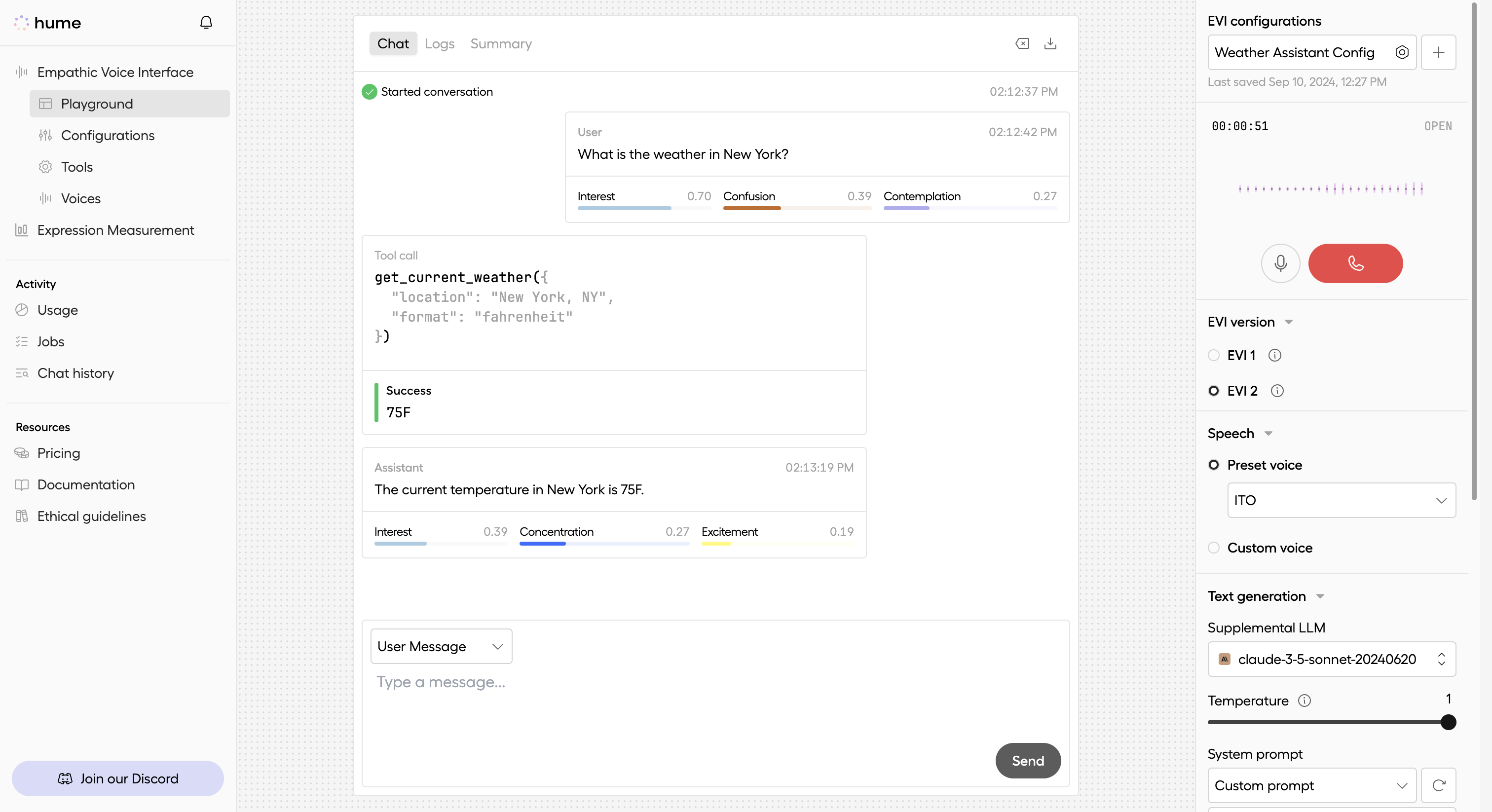
To summarize, Tool Call serves as a programmatic tool for intelligently signaling when you should invoke your function. EVI does not invoke the function for you. You will need to define a function, invoke the function, and pass the return value of your function to EVI via a Tool Response message. EVI will generate a response based on the content of your message.
Using built-in tools
User-defined tools allow EVI to identify when a function should be invoked, but you will need to invoke the function itself. On the other hand, Hume also provides built-in tools that are natively integrated. This means that you don’t need to define the function; EVI handles both determining when the function needs to be called and invoking it.
Hume supports the following built-in tools:
- web_search: Enables EVI to search the web for real-time information when needed.
- hang_up: Closes the WebSocket connection with status code
1000
when appropriate (e.g., after detecting a farewell, signaling the end of the conversation).
This section explains how to specify built-in tools in your configurations and details the message flow you can expect when EVI uses a built-in tool during a chat session.
Specify built-in tool in EVI configuration
Let’s begin by creating a configuration which includes the built-in web search tool. To specify the web search tool in your EVI configuration, during the Add tools step, ensure Web search is enabled. Refer to our Configuration Guide for more details on creating a configuration.
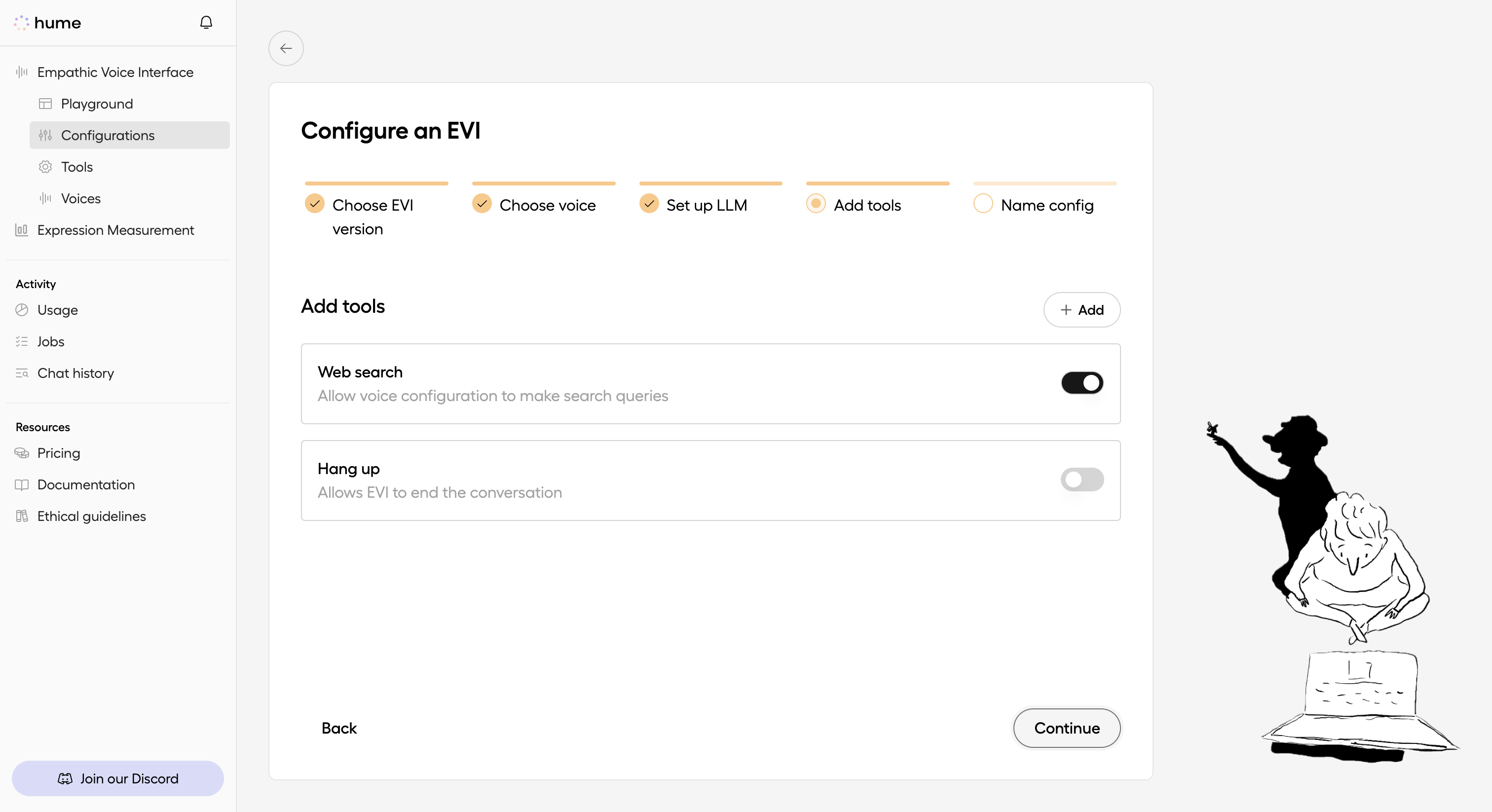
Alternatively, you can specify the built-in tool by making a POST request to /configs with the following request body:
Upon success, expect EVI to return a response similar to this example:
EVI uses built-in tool
Now that we’ve created an EVI configuration which includes the built-in web search tool, let’s test it out in the EVI Playground. Try asking EVI a question that requires web search, like “what is the latest news with AI research?”
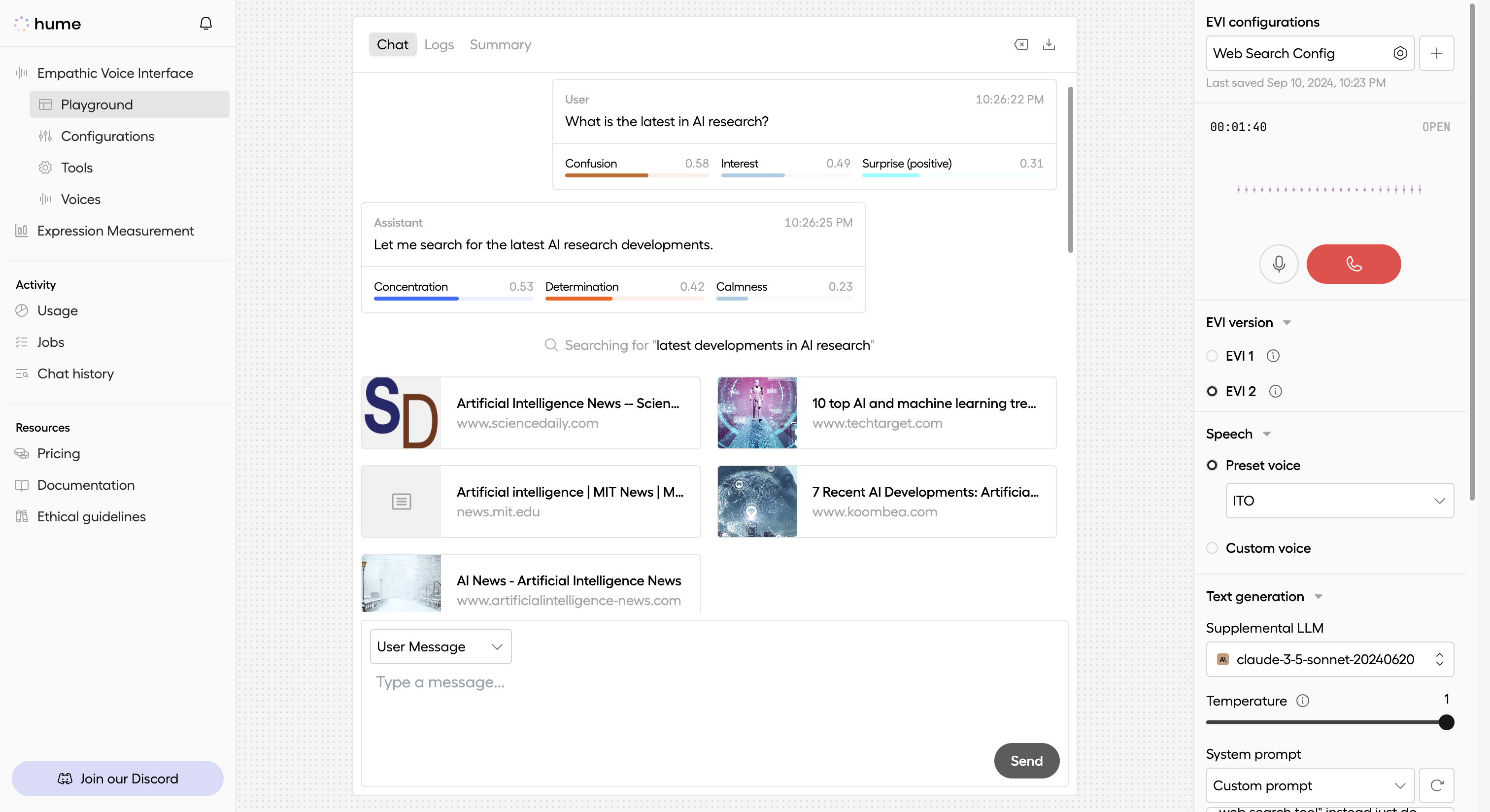
EVI will send a response generated from the web search results:
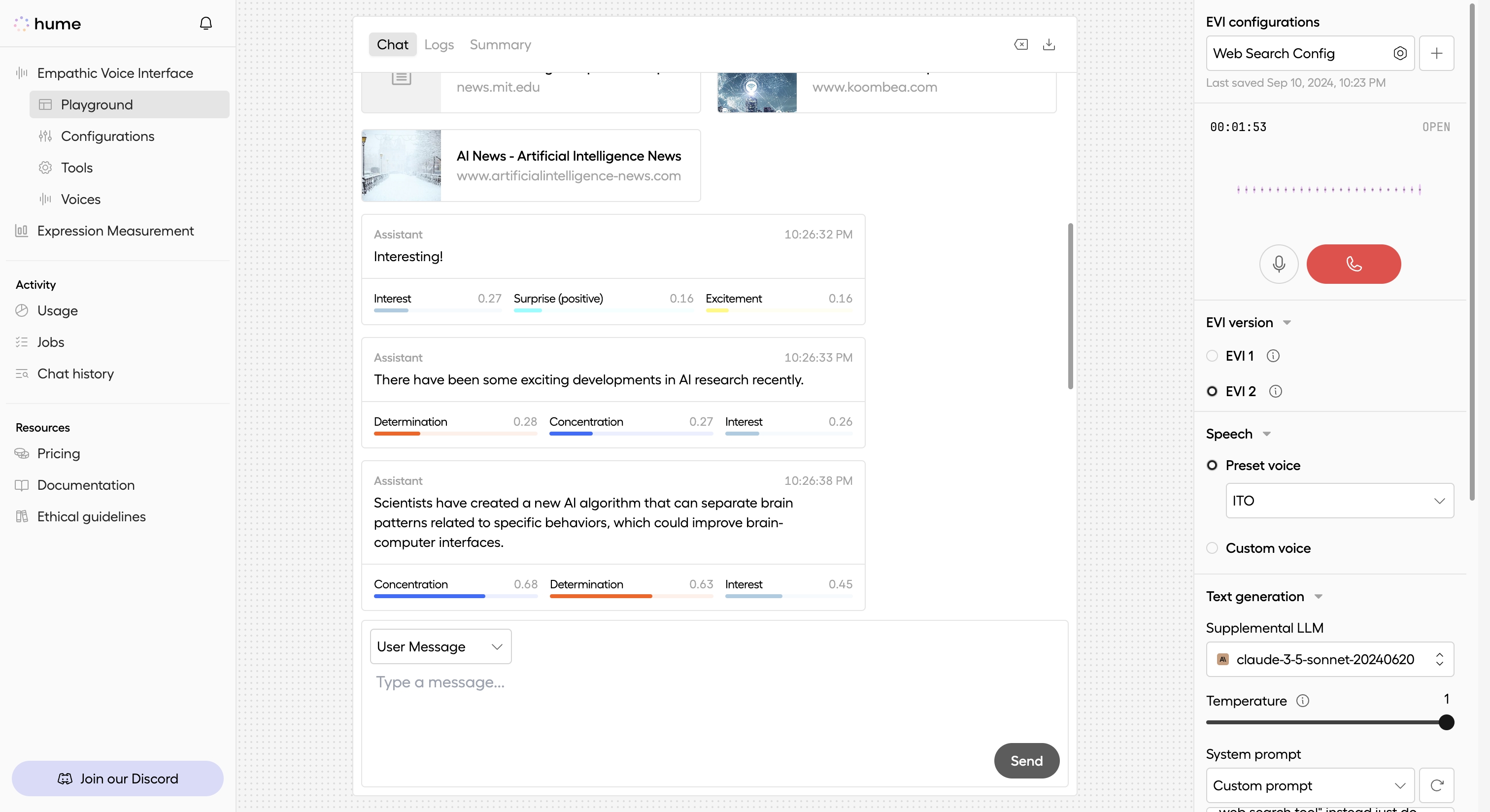
Let’s review the message flow for when web search is invoked.
Interruptibility
Function calls can be interrupted to cancel them or to resend them with updated parameters.
Canceling a function call
Just as EVI is able to infer when to make a function call, it can also infer from the user’s input when to cancel one. Here is an overview of what the message flow would look like:
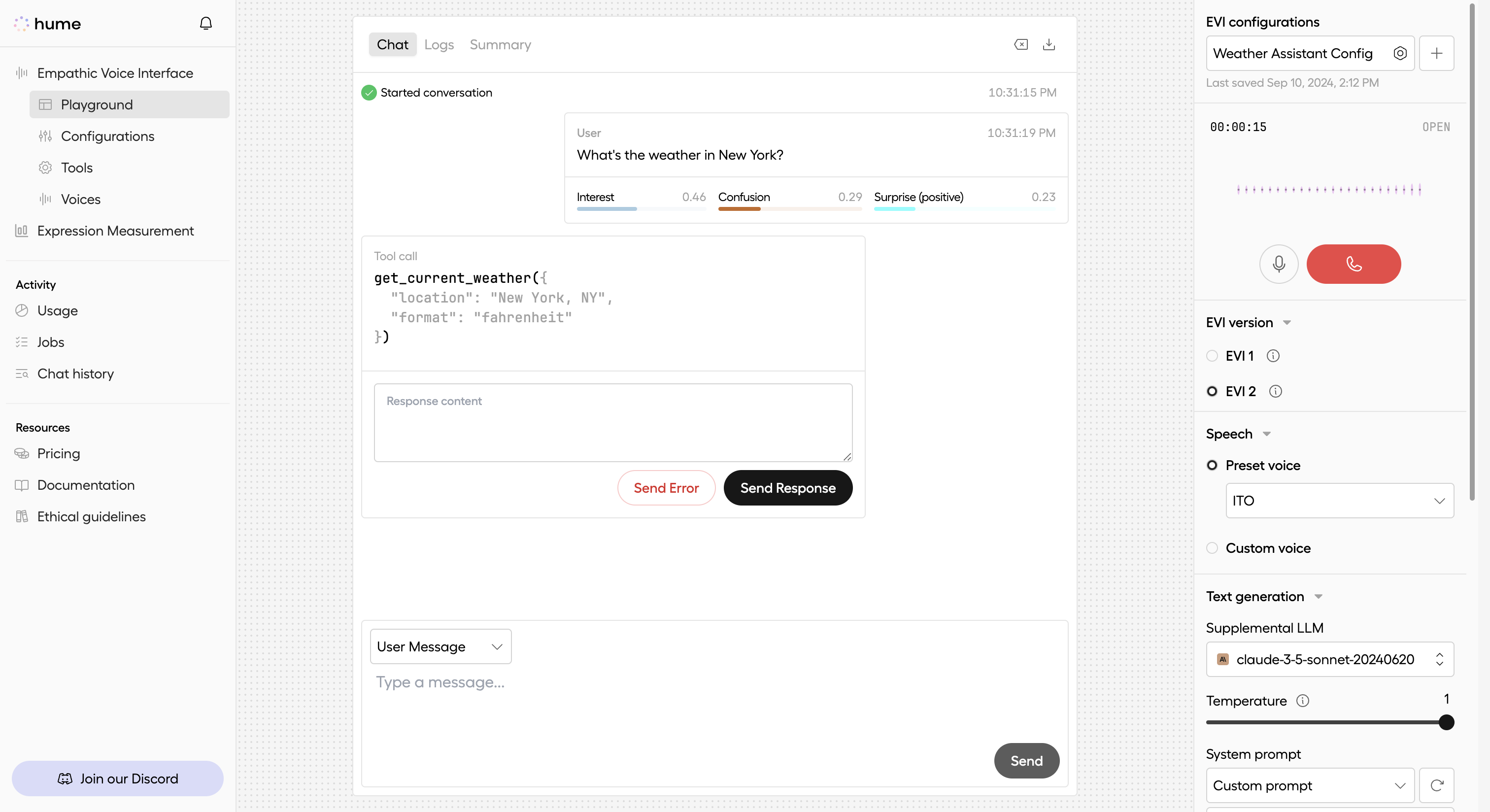
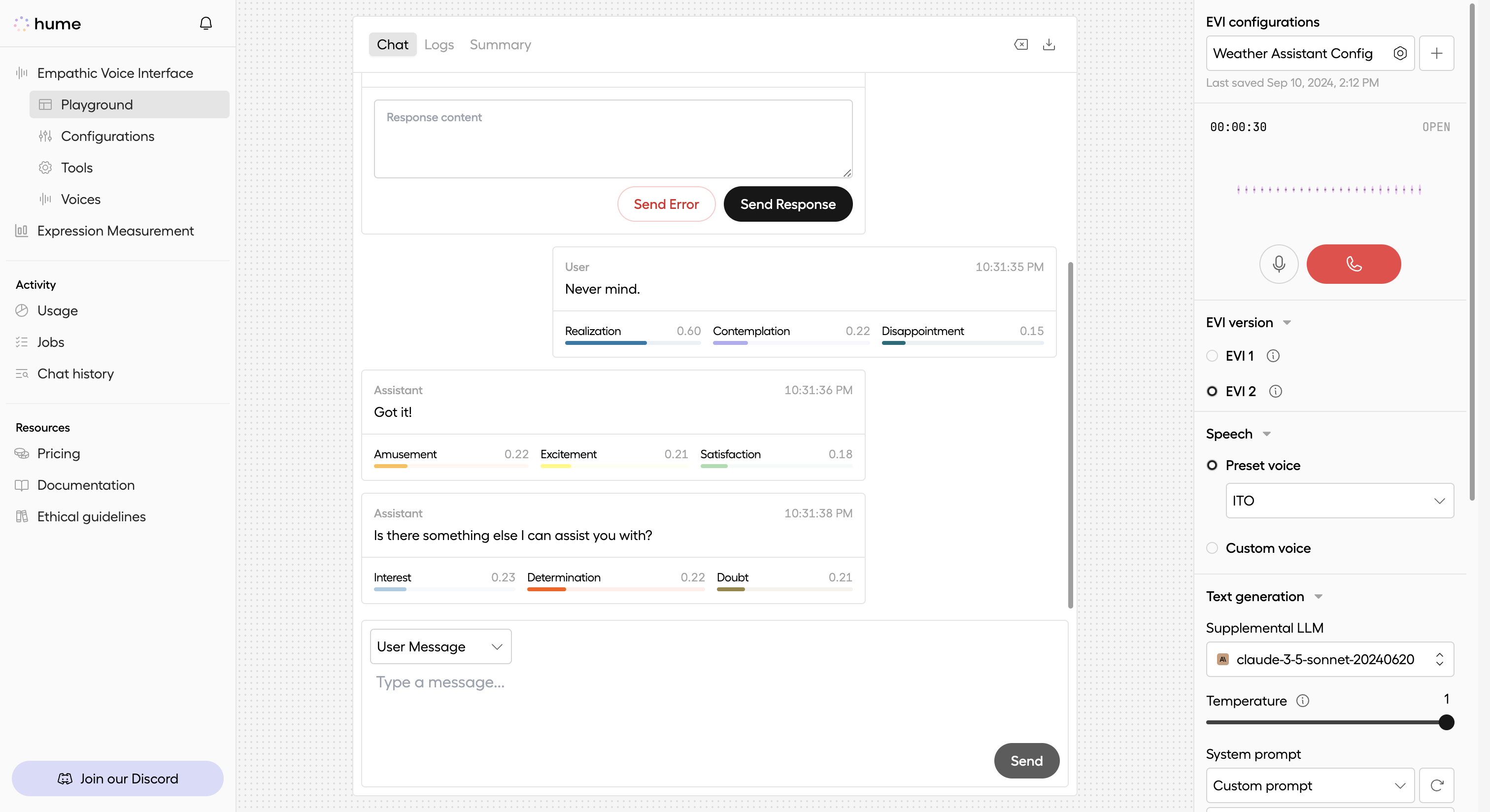
Updating a function call
Sometimes we don’t necessarily want to cancel the function call, and instead want to update the parameters. EVI can infer the difference. Below is a sample flow of interrupting the interface to update the parameters of the function call:
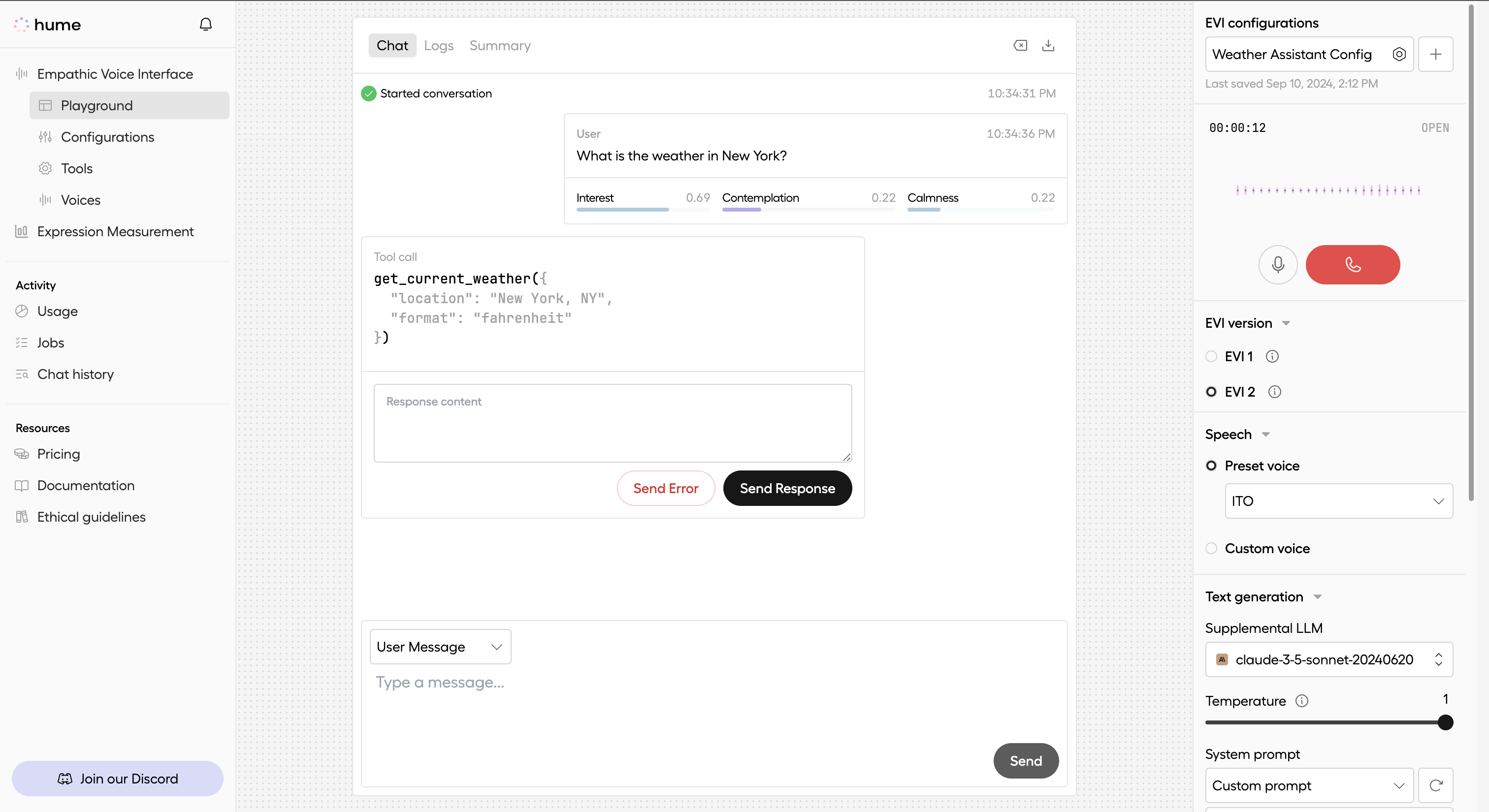
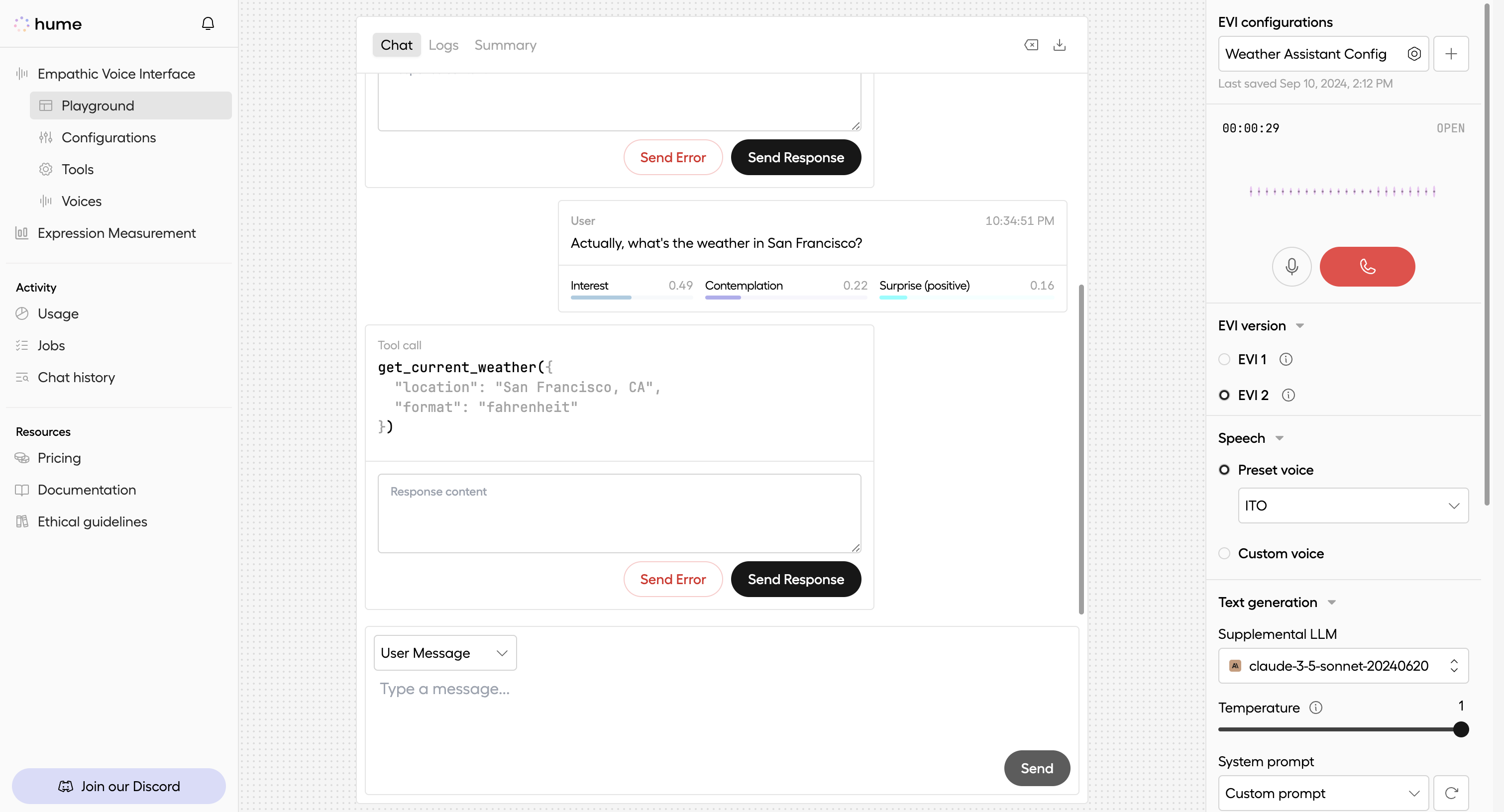
Handling errors
It’s possible for tool use to fail. For example, it can fail if the Tool Response message content was not in UTF-8 format or if the function call response timed out. This section outlines how to specify fallback content to be used by EVI to communicate a failure, as well as the message flow for when a function call failure occurs.
Specifying fallback content
When defining your Tool, you can specify fallback content within the Tool’s fallback_content
field. When the Tool
fails to generate content, the text in this field will be sent to the LLM in place of a result. To accomplish this,
let’s update the Tool we created during setup to include fallback content. We can accomplish this by publishing a new
version of the Tool via a POST request to /tools/{id}:
Failure message flow
This section outlines the sort of messages that can be expected when Tool use fails. After sending a Tool Response message, we will know an error, or failure, occurred when we receive the Tool Error message:
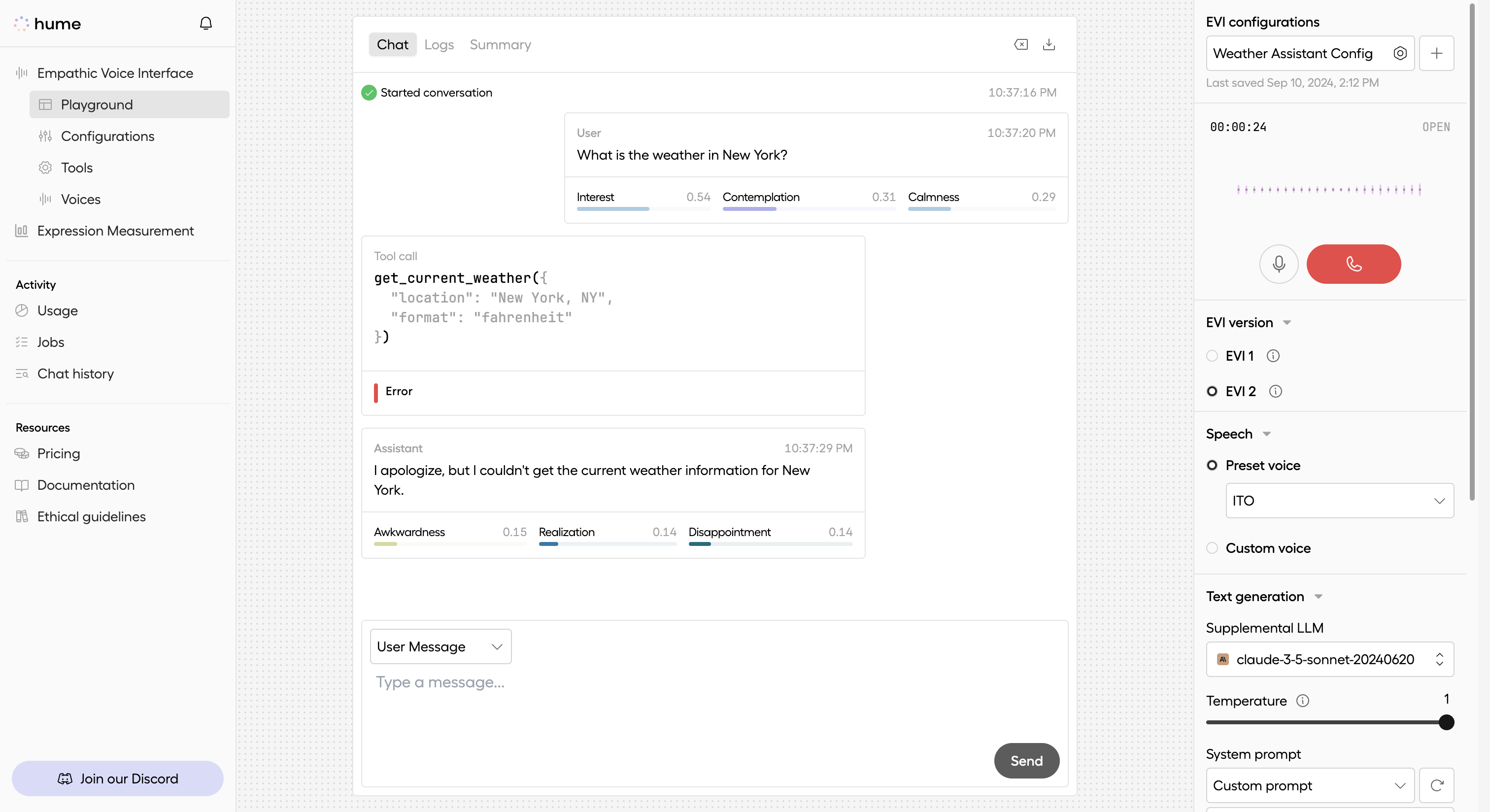
Let’s cover another type of failure scenario: what if the weather API the function was using was down? In this case,
we would send EVI a Tool Error
message. When sending the Tool Error message, we can specify fallback_content
to be more specific to the error
our function throws. This is what the message flow would be for this type of failure:
Let’s revisit our function for handling Tool Call messages from the Function Calling section. We can now add support for error handling by sending Tool Error messages to EVI. This will enable our function to handle cases where fetching the weather fails or the requested tool is not found: